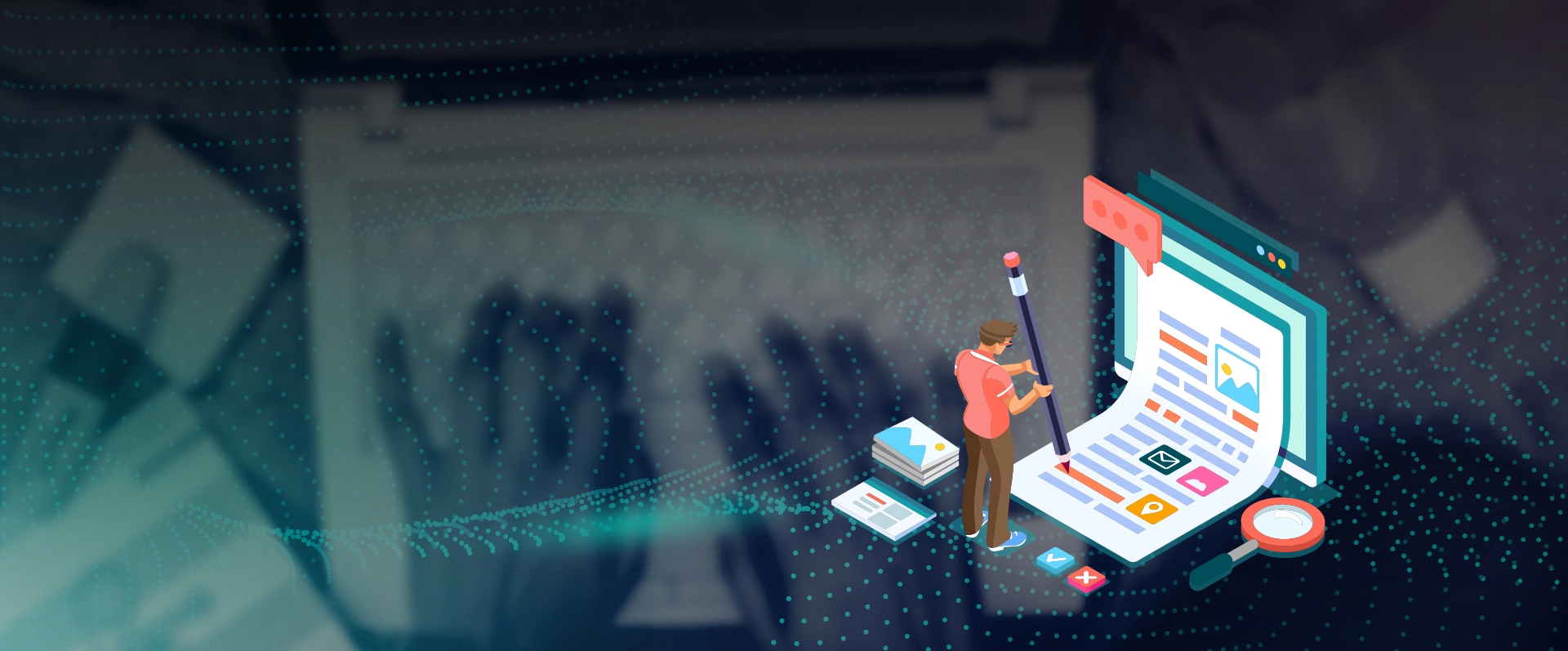
Microservices in Java - Spring Cloud and Netflix Overview - Part 1
As it is well known, there is a lot of momentum around microservices. The transition from monolithic to microservice-based architecture gives many future benefits in terms of maintainability, scalability, high availability, etc. In the following text, we will talk about Spring Cloud.
What are Microservices?
A microservice, also known as the microservice architecture, is an architectural style that structures an application as a collection of services. Benefits of the microservice architecture are as follows:
- Small modules
- Loosely coupled
- Independently deployable
- Faster defect isolation
The microservice architecture enables the rapid, frequent, and reliable delivery of large, complex applications. It also enables an organization to evolve its technology stack. Apart from this, the microservice architecture helps us to achieve continuous delivery and integration.
What is Spring Cloud and What is It Used For?
Spring Cloud is a framework for building robust cloud applications and it provides a solution to the commonly encountered patterns when developing a distributed system. Spring Cloud framework provides tools for developers to quickly build both cloud applications and microservice-based applications.
Using Spring Cloud, a developer can quickly develop services and applications that implement the design patterns that work well in any distributed environment. However, Spring Cloud is not a single-unit project, but a group of projects for managing the challenges of development of cloud-native systems. Some important projects are the following:
- Spring Cloud Config
- Spring Cloud Netflix
- Spring Cloud Bus
- Spring Cloud Sleuth
- Spring Cloud Kubernetes
Spring Cloud Netflix is the most popular project that is part of Spring Cloud. It has around 3.5k stars on GitHub. It provides Netflix OSS integrations for Spring Boot apps through auto-configuration and binding to the Spring Environment. With a few simple annotations, you can quickly enable and configure the common patterns inside your application and start building large distributed systems.


Service Discovery – Netflix Eureka
In distributed computing, there is a concept called Service Discovery and Registration where one dedicated server is responsible for maintaining the registry of all the microservices that have been deployed and removed.
Think of it as a lookup service where microservices (clients) can register themselves and discover other registered microservices. When a client microservice registers with Eureka, it provides metadata such as host, post, and health indicators thus allowing other microservices to discover it. The discovery server expects a regular heartbeat message from each microservice instance. If an instance begins to consistently fail to send a heartbeat, the discovery server will remove the instance from its registry. This way we will have a very stable ecosystem of microservices collaborating with each other. Also, we don’t have to manually maintain the address of other microservices, which is a next to impossible task if the scale up or down is very frequent and we use a virtual host to host the services, especially in the cloud environment.
To create Eureka server, we need to add Eureka Server dependency and annotate Spring Boot’s main class with @EnableEurekaServer.
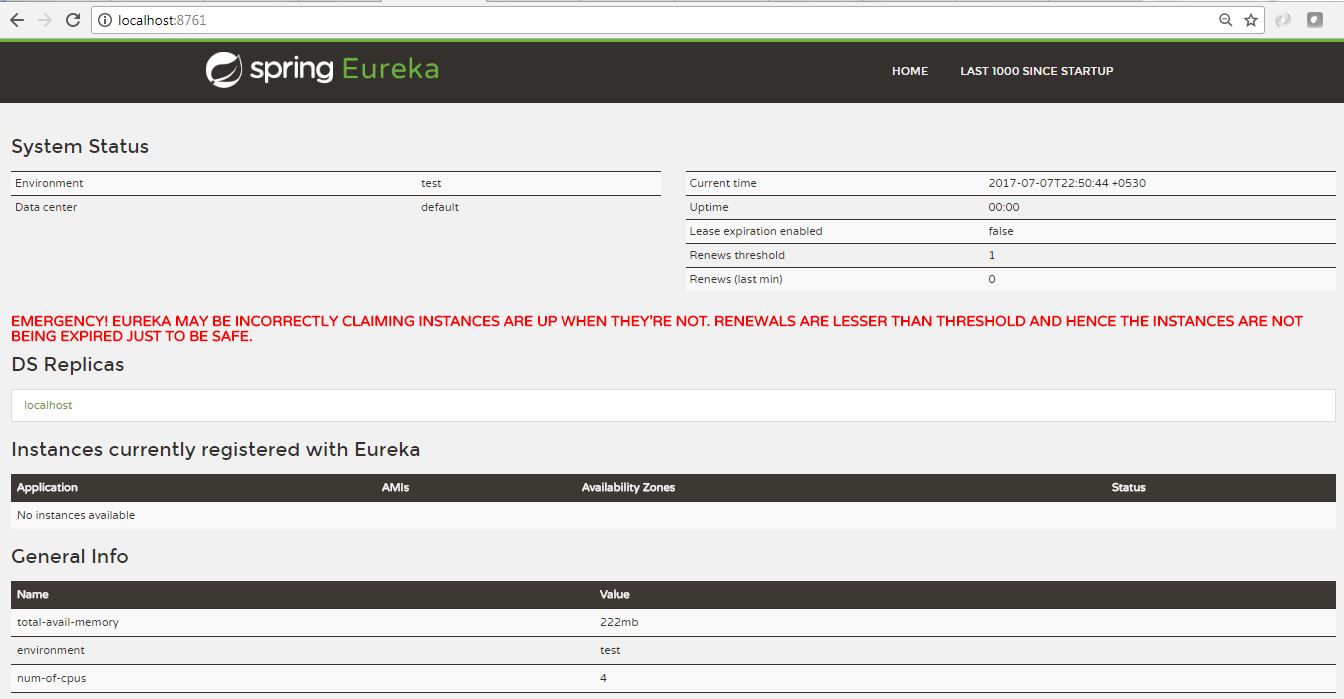
In this case, no service is registered here which is expected and once we spin up the client services, this server will update with the details of the client services. When we want to register a new service instance with the Eureka Server, we need to have Eureka Discovery Client dependency and to annotate Spring Boot’s main class with @EnableEurekaClient.
Edge Server – Netflix Zuul
Zuul is the front door for all requests from devices and websites to the backend. As an edge service application, Zuul is built to enable dynamic routing, monitoring, resiliency, and security. Routing is an important part of a microservice architecture.
To create Eureka server, we need to add Eureka Server dependency and annotate Spring Boot’s main class with @EnableEurekaServer.

Zuul also provides a well-known entry point to the microservices in the system. Using dynamically allocated ports is convenient to avoid port conflicts and to minimize administration, but it makes it harder for any given service consumer.
In order to build a Zuul gateway, the microservice that handles the UI needs to be enabled with zuul config. We have to add spring-cloud-starter-zuul dependency and enable it in Spring Boot’s main class using @EnableZuulProxy annotation. The advantage of this type of design is that those common aspects like CORS, authentication, and security can be put into a centralized service so that all common aspects are applied on each request. Furthermore, with Zuul, we can implement any routing rules or any filter implementation.

Zuul has mainly four types of filters that enable us to intercept the traffic in a different timeline of the request processing for any particular transaction. We can add any number of filters for a particular URL pattern.
- Pre filters are invoked before the request is routed
- Post filters are invoked after the request has been routed
- Route filters are used to route the request
- Error filters are invoked when an error occurs while handling the request
Conclusion – Part 1
The main objective of the microservices implementation is to split up the application as a separate service for each core and API service functionality. In Part 1, we have introduced the microservices architecture and the Spring Cloud project. Also, we have talked about two important components of the Spring Cloud Project – Eureka Service Discovery and Zuul Edge Server.
But that is not all, in Part 2 we will continue our talk about microservices and introduce the other three components.
FAQ
Spring Cloud provides a comprehensive set of tools for building scalable and resilient microservices, including service discovery, distributed configuration, circuit breakers, and centralized logging. It simplifies the management of complex microservices architectures.
Netflix Zuul acts as an API Gateway in a microservices ecosystem, handling tasks such as routing, authentication, load balancing, and request filtering. It serves as a single entry point for managing client requests and forwarding them to appropriate microservices.
Common challenges include managing inter-service communication, ensuring data consistency across distributed services, handling service failures gracefully, and monitoring the overall system health. Proper design patterns and tools like Spring Cloud Config, Hystrix, and Sleuth help address these issues.